Consent, Authorization, and SCA
Introduction
Under the PSD2 directive, banks can require Strong Customer Authentication (SCA) of requests for account data or payment initiations on behalf of third parties (i.e., Neonomics clients). When interacting with the Neonomics API, your application will need to handle SCA requests to get account data and make payments. This guide takes you through how SCA works in the Neonomics API.
Two forms of SCA exist within PSD2 and the Neonomics API: consent and payment authorization.
What is consent
Consent allows a third party to access an end-user's account to get account information or initiate payments.
When an end-user gives consent to account access, it remains valid for a specific period. The validity period is normally 90 days. End-users can also choose to revoke their consent at any time through their online banking service. As long as a valid consent exists, the platform will not require a new one.
According to an EBA opinion (Opinion of the European Banking Authority on obstacles under Article 32(3) of the RTS on SCA and CSC, article 24), consent shall only be required for account information requests. However, some banks still require consent for payment initiation requests.
How to handle consent
When you intend to make a request for either payment initiation or account information, you cannot know in advance if the bank will require consen_t for it. That means you first have to attempt the request and then handle_consent if necessary.
If the bank requires consent, the Neonomics API will return an error object with errorCode 1426. You will then have to instruct the end-user to complete SCA.
The first step is to call Get Consent API. The error object contains data needed to populate the request parameters, including the URL. The response from that call will include the consent URL.
The next step is to present the consent URL to the end-user so they can complete SCA to give consent to account access.
Once the end-user has given consent, you can request account data or initiate a payment for that end-user.
Please see How to get an end-user's consent below for a detailed example of consent handling.
Info
Please do not call the /consent endpoint unless provided with a link from the 1426 error. Our platform automatically tracks the need for consent and informs you when a bank requires consent for an operation
What is authorization
An authorization is the end-user’s approval of the initiation of one specific payment. This authorization requires Strong Customer Authentication (SCA). Payment authorization can be requested for each payment initiation, unlike consent, which needs to be renewed at regular intervals.
Low-risk payments are exempt from authorization. The account owner's bank usually decides what payment types to consider as low risk. Example: The account owner can initiate five payments below €30 without requiring authorization.
How to handle authorization
When you intend to initiate a payment, you cannot know in advance if the bank will require authorization of it or not. That means you first have to initiate the payment and then handle authorization if necessary.
When the bank requires payment authorization, the Neonomics API will return an error object with errorCode 1428. You will then have to instruct the end-user to complete SCA.
The first step is to call Authorize Payment API. The error object contains data needed to populate the request parameters, including the URL. The response from that call will include the authorization URL.
The next step is to present the authorization URL to the end-user so they can complete SCA to authorize the payment.
As soon as the end-user has authorized the payment, you have to call Complete Payment API.
Please see the payments guides for detailed examples of the payment flow and how to handle authorization.
Info
Please do not call the /authorize endpoint unless provided with a link from the 1428 error. Our platform automatically tracks the need for authorization and informs you when a bank requires it for an operation.
SCA - Strong Customer Authentication
SCA is also called multi-factor authentication. It’s based on the use of two or more elements categorized as:
- knowledge: something only the user knows, e.g., password, PIN code
- possession: something only the user possesses, e.g., smartphone, code generator
- inherence: something the user is, e.g., fingerprint, facial recognition
Decoupled SCA
The Neonomics platform supports SCA in decoupled flows. End-users will then be prompted with an authorization flow on their device, for example, with a QR code or bankid on mobile.
Redirect end-user after SCA
When end-users have completed SCA, the flow is redirected to a specific URL. This callback can happen in two ways:
- Standard redirection to a URL provided by Neonomics, which is the default option.
- Custom redirection to a custom URL provided by the client's application.
The custom URL must first be registered for the relevant application in your Dashboard in the Developer Portal. You can enter up to three Redirect URL(s) per application.
When calling Consent API, include the x-redirect-url parameter in the header, using the value of the custom URL.
Info
We recommend that you not build your implementation of the redirect flow around the use of iFrames. It will work in our sandbox, but several banks have restrictions in force that will cause problems.
How to get an end-user's consent
This guide uses the Accounts API endpoint, but a similar flow can also be used for a payment API request. To get an end-user’s consent, follow these three steps:
- Try to fetch accounts data using the accounts endpoint, to get a 1426 error.
- Get the consent URL from the banks using the consent endpoint.
- Get the end-user to give their consent using the URL provided by the bank.
Please note that the bank details used in this document are test details used only in the sandbox.
Info
You need to complete the Development quickstart guide before starting this guide. A valid
access_token
and sessionId are needed to follow this guide.
Step 1: Try to fetch account data
The first step is an attempt to request an end-user's account data.
To call Accounts API, make a GET
request to the /accounts/ endpoint. This endpoint is used to get all the accounts associated with a session. A sessionId
is the link between an end-user and a bank.
Use the curl GET
request below to fetch the accounts. Replace \<ACCESS_TOKEN>
and \<SESSION_ID>
with access_token
and sessionId
that was generated previously, for example when going through the the Quickstart guide.
Request:
curl -X GET <https://sandbox.neonomics.io/ics/v3/accounts>
-H "Authorization:Bearer \<ACCESS_TOKEN>"
-H "x-device-id:example_id_for_quickstart"
-H "Accept:application/json"
-H "x-psu-ip-address:109.74.179.3"
-H "x-session-id:\<SESSION_ID>"
Below is the 1426
error returned by the Accounts API endpoint when consent is required:
Response:
{
"id": "4d2f1c14-8d48-47b6-a499-2e75581fce64",
"errorCode": "1426",
"message": "This resource needs to initialise consent. Please use the URL provided to continue. The consent Url will provide the bank authentication url. You can consume this resource after authenticating.",
"source": "C",
"type": "CONSENT",
"timestamp": 1582028491292,
"links": [
{
"type": "GET",
"rel": "consent",
"href": "https://development-api.neonomics.io/ics/v3/consent/d83a5db5-4dd9-4596-be50-708fb72de89a?scope=accounts",
"meta":
{
"id": "d83a5db5-4dd9-4596-be50-708fb72de89a"
}
}
]
}
Link object
Neonomics API errors can contain an array of link objects. The error shown above contains a link object with the following attributes:
- href - This attribute can contain two types of links: The first type is the URL for a request to the Neonomics API as in the example above. The second type is a link to the bank's website so the end-user can complete a consent request or payment authorization.
- rel - Name of the Neonomics API operation related to the link.
- type - If the href is a link to a request to the Neonomics API, then this is the type of HTTP RESTful request method required:
POST
,GET
,DELETE
,PUT
andPATCH
. - meta - This object contains an id which is the current
sessionId
.
Save the href you received from the request above, as you will need it in step 2.
Step 2: Get the consent URL
Now you have received a 1426 error, and to get the consent URL from the bank, you will need to call the Consent API using the GET
curl request below.
You will need the URL you obtained in the error object in step one. Replace \<CONSENT_URL>
with the href obtained in step one. Replace \<ACCESS_TOKEN>
with your access_token
.
Request:
curl -X GET \<CONSENT_URL>
-H "Authorization:Bearer \<ACCESS_TOKEN>"
-H "Accept:application/json"
-H "x-device-id:example_id_for_quickstart"
-H "x-psu-ip-address:109.74.179.3"
In cases where you need to apply a Redirect URL, include the x-redirect-url parameter in the request.
Below is the response returned from the request above.
Response:
{
"message": "Please consent with the bank authentication URL provided to continue.",
"links": [
{
"type": "GET",
"rel": "consent",
"href": "https://openbanking.sbanken.no/tap-sandbox/9710/?route_secesb_id=1&flow=psd2&state=63cd8a59-174a-406f-a1bb-8d359d149617"
}
]
}
This response contains an array of links that has one object. The href in this object is a link for the end-user to complete customer consent.
Save the href URL, as you will need it in the next step.
Step 3: Get the end-user to give their consent
To complete the steps in this guide, obtain end-user consent. In this example you will act as the end-user. Copy the href URL from the previous step and open it in your internet browser. Below is a sandbox example of the consent webpage for the Norwegian bank S'banken.
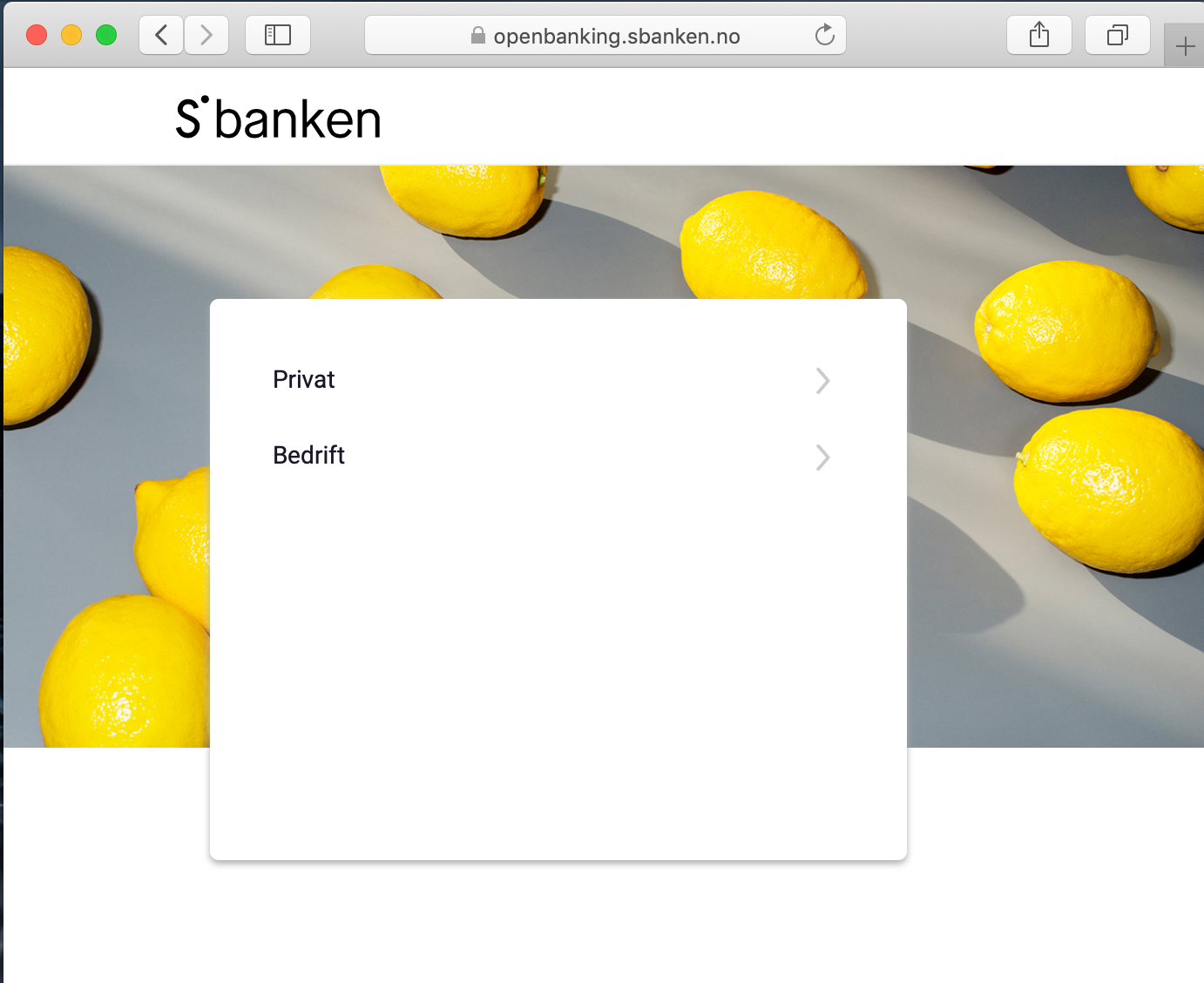
Click ‘Privat’, then page below will open. Here the end-user will be asked to provide a ‘Fødselsnummer’. Enter this number: 13039319955
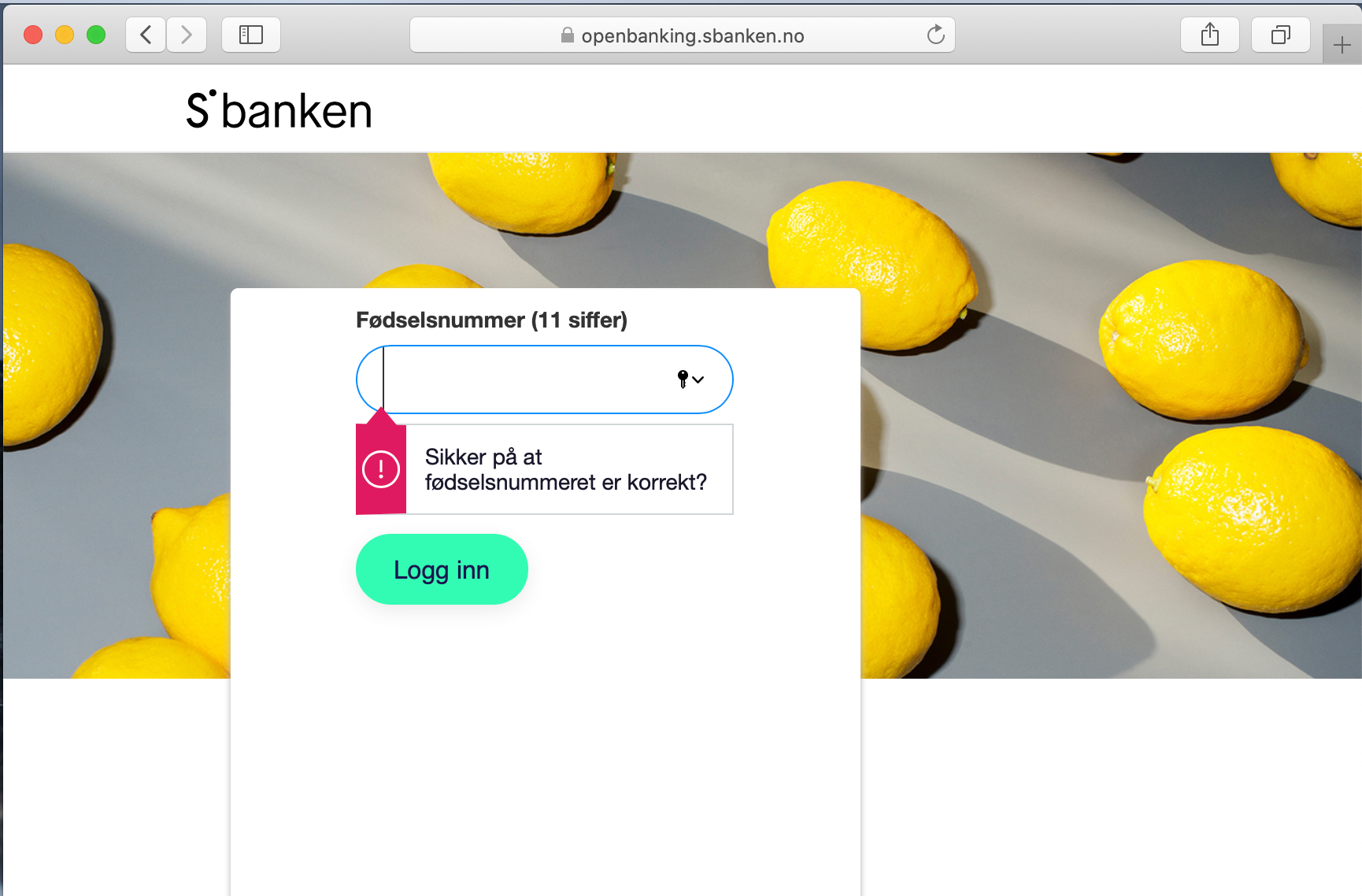
The next page is shown below, make sure all checkboxes are ticked, then click 'Bekreft'.
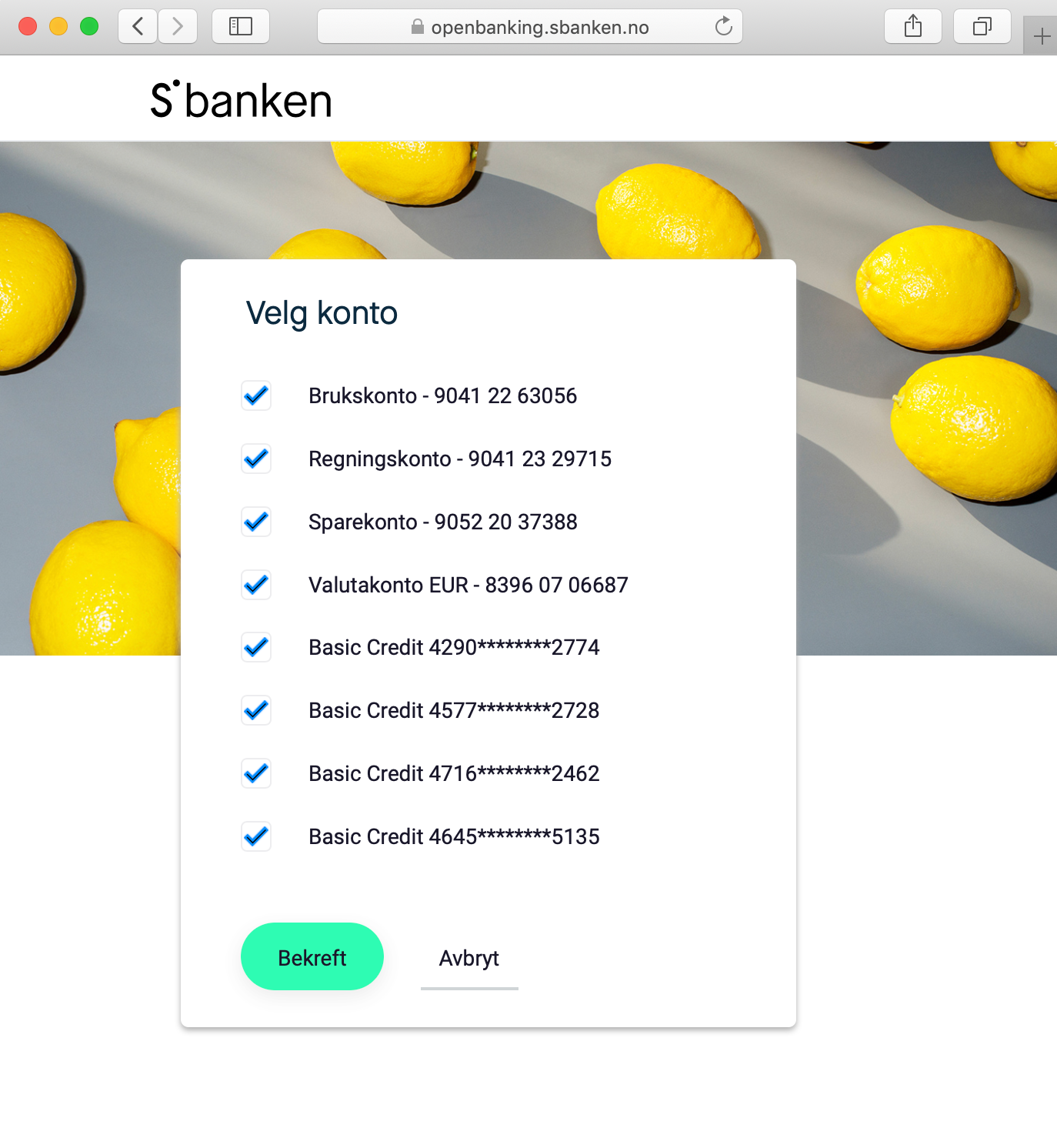
This will redirect you to the Neonomics webpage below. However, if a custom Redirect URL was applied in the previous step, the page specified in x-redirect-url will appear instead.
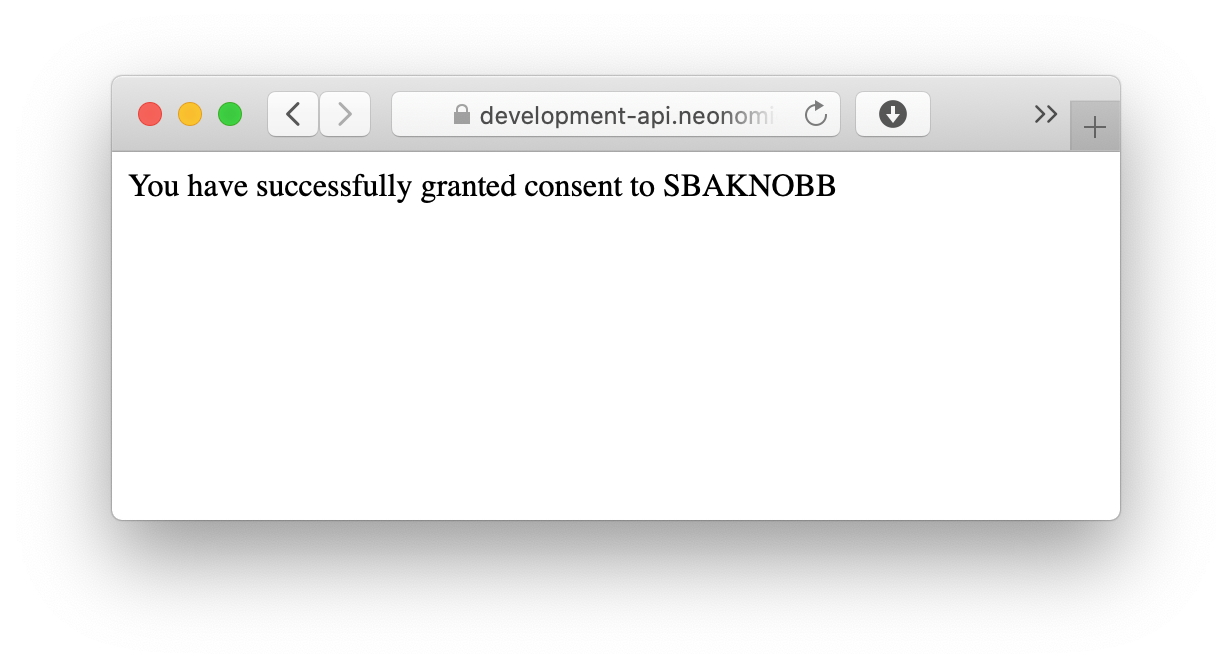
You have now granted end-user consent for your sessionId
. Great work! 🦞
Updated 2 months ago